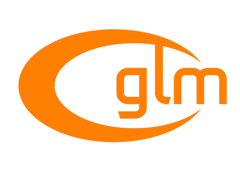 |
0.9.7
|
Go to the documentation of this file.
281 #if(GLM_COMPILER & GLM_COMPILER_GCC)
283 # if(GLM_COMPILER >= GLM_COMPILER_GCC43)
284 # define GLM_CXX11_STATIC_ASSERT
287 #elif(GLM_COMPILER & (GLM_COMPILER_APPLE_CLANG | GLM_COMPILER_LLVM))
288 # if(__has_feature(cxx_exceptions))
289 # define GLM_CXX98_EXCEPTIONS
292 # if(__has_feature(cxx_rtti))
293 # define GLM_CXX98_RTTI
296 # if(__has_feature(cxx_access_control_sfinae))
297 # define GLM_CXX11_ACCESS_CONTROL_SFINAE
300 # if(__has_feature(cxx_alias_templates))
301 # define GLM_CXX11_ALIAS_TEMPLATE
304 # if(__has_feature(cxx_alignas))
305 # define GLM_CXX11_ALIGNAS
308 # if(__has_feature(cxx_attributes))
309 # define GLM_CXX11_ATTRIBUTES
312 # if(__has_feature(cxx_constexpr))
313 # define GLM_CXX11_CONSTEXPR
316 # if(__has_feature(cxx_decltype))
317 # define GLM_CXX11_DECLTYPE
320 # if(__has_feature(cxx_default_function_template_args))
321 # define GLM_CXX11_DEFAULT_FUNCTION_TEMPLATE_ARGS
324 # if(__has_feature(cxx_defaulted_functions))
325 # define GLM_CXX11_DEFAULTED_FUNCTIONS
328 # if(__has_feature(cxx_delegating_constructors))
329 # define GLM_CXX11_DELEGATING_CONSTRUCTORS
332 # if(__has_feature(cxx_deleted_functions))
333 # define GLM_CXX11_DELETED_FUNCTIONS
336 # if(__has_feature(cxx_explicit_conversions))
337 # define GLM_CXX11_EXPLICIT_CONVERSIONS
340 # if(__has_feature(cxx_generalized_initializers))
341 # define GLM_CXX11_GENERALIZED_INITIALIZERS
344 # if(__has_feature(cxx_implicit_moves))
345 # define GLM_CXX11_IMPLICIT_MOVES
348 # if(__has_feature(cxx_inheriting_constructors))
349 # define GLM_CXX11_INHERITING_CONSTRUCTORS
352 # if(__has_feature(cxx_inline_namespaces))
353 # define GLM_CXX11_INLINE_NAMESPACES
356 # if(__has_feature(cxx_lambdas))
357 # define GLM_CXX11_LAMBDAS
360 # if(__has_feature(cxx_local_type_template_args))
361 # define GLM_CXX11_LOCAL_TYPE_TEMPLATE_ARGS
364 # if(__has_feature(cxx_noexcept))
365 # define GLM_CXX11_NOEXCEPT
368 # if(__has_feature(cxx_nonstatic_member_init))
369 # define GLM_CXX11_NONSTATIC_MEMBER_INIT
372 # if(__has_feature(cxx_nullptr))
373 # define GLM_CXX11_NULLPTR
376 # if(__has_feature(cxx_override_control))
377 # define GLM_CXX11_OVERRIDE_CONTROL
380 # if(__has_feature(cxx_reference_qualified_functions))
381 # define GLM_CXX11_REFERENCE_QUALIFIED_FUNCTIONS
384 # if(__has_feature(cxx_range_for))
385 # define GLM_CXX11_RANGE_FOR
388 # if(__has_feature(cxx_raw_string_literals))
389 # define GLM_CXX11_RAW_STRING_LITERALS
392 # if(__has_feature(cxx_rvalue_references))
393 # define GLM_CXX11_RVALUE_REFERENCES
396 # if(__has_feature(cxx_static_assert))
397 # define GLM_CXX11_STATIC_ASSERT
400 # if(__has_feature(cxx_auto_type))
401 # define GLM_CXX11_AUTO_TYPE
404 # if(__has_feature(cxx_strong_enums))
405 # define GLM_CXX11_STRONG_ENUMS
408 # if(__has_feature(cxx_trailing_return))
409 # define GLM_CXX11_TRAILING_RETURN
412 # if(__has_feature(cxx_unicode_literals))
413 # define GLM_CXX11_UNICODE_LITERALS
416 # if(__has_feature(cxx_unrestricted_unions))
417 # define GLM_CXX11_UNRESTRICTED_UNIONS
420 # if(__has_feature(cxx_user_literals))
421 # define GLM_CXX11_USER_LITERALS
424 # if(__has_feature(cxx_variadic_templates))
425 # define GLM_CXX11_VARIADIC_TEMPLATES
428 #endif//(GLM_COMPILER & (GLM_COMPILER_APPLE_CLANG | GLM_COMPILER_LLVM))