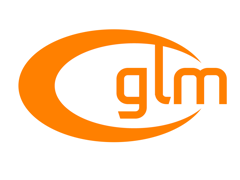 |
0.9.8
|
Go to the documentation of this file.
16 #include "../detail/type_vec2.hpp"
17 #include "../detail/type_vec3.hpp"
18 #include "../detail/type_vec4.hpp"
19 #include "../detail/type_mat2x2.hpp"
20 #include "../detail/type_mat2x3.hpp"
21 #include "../detail/type_mat2x4.hpp"
22 #include "../detail/type_mat3x2.hpp"
23 #include "../detail/type_mat3x3.hpp"
24 #include "../detail/type_mat3x4.hpp"
25 #include "../detail/type_mat4x2.hpp"
26 #include "../detail/type_mat4x3.hpp"
27 #include "../detail/type_mat4x4.hpp"
28 #include "../gtc/quaternion.hpp"
29 #include "../gtx/dual_quaternion.hpp"
31 #if GLM_MESSAGES == GLM_MESSAGES_ENABLED && !defined(GLM_EXT_INCLUDED)
32 # pragma message("GLM: GLM_GTX_type_trait extension included")
40 template <
template <
typename, precision>
class genType,
typename T, precision P>
43 static bool const is_vec =
false;
44 static bool const is_mat =
false;
45 static bool const is_quat =
false;
46 static length_t
const components = 0;
47 static length_t
const cols = 0;
48 static length_t
const rows = 0;
51 template <
typename T, precision P>
52 struct type<tvec1, T, P>
54 static bool const is_vec =
true;
55 static bool const is_mat =
false;
56 static bool const is_quat =
false;
63 template <
typename T, precision P>
64 struct type<tvec2, T, P>
66 static bool const is_vec =
true;
67 static bool const is_mat =
false;
68 static bool const is_quat =
false;
75 template <
typename T, precision P>
76 struct type<tvec3, T, P>
78 static bool const is_vec =
true;
79 static bool const is_mat =
false;
80 static bool const is_quat =
false;
87 template <
typename T, precision P>
88 struct type<tvec4, T, P>
90 static bool const is_vec =
true;
91 static bool const is_mat =
false;
92 static bool const is_quat =
false;
99 template <
typename T, precision P>
100 struct type<tmat2x2, T, P>
102 static bool const is_vec =
false;
103 static bool const is_mat =
true;
104 static bool const is_quat =
false;
113 template <
typename T, precision P>
114 struct type<tmat2x3, T, P>
116 static bool const is_vec =
false;
117 static bool const is_mat =
true;
118 static bool const is_quat =
false;
127 template <
typename T, precision P>
128 struct type<tmat2x4, T, P>
130 static bool const is_vec =
false;
131 static bool const is_mat =
true;
132 static bool const is_quat =
false;
141 template <
typename T, precision P>
142 struct type<tmat3x2, T, P>
144 static bool const is_vec =
false;
145 static bool const is_mat =
true;
146 static bool const is_quat =
false;
155 template <
typename T, precision P>
156 struct type<tmat3x3, T, P>
158 static bool const is_vec =
false;
159 static bool const is_mat =
true;
160 static bool const is_quat =
false;
169 template <
typename T, precision P>
170 struct type<tmat3x4, T, P>
172 static bool const is_vec =
false;
173 static bool const is_mat =
true;
174 static bool const is_quat =
false;
183 template <
typename T, precision P>
184 struct type<tmat4x2, T, P>
186 static bool const is_vec =
false;
187 static bool const is_mat =
true;
188 static bool const is_quat =
false;
197 template <
typename T, precision P>
198 struct type<tmat4x3, T, P>
200 static bool const is_vec =
false;
201 static bool const is_mat =
true;
202 static bool const is_quat =
false;
211 template <
typename T, precision P>
212 struct type<tmat4x4, T, P>
214 static bool const is_vec =
false;
215 static bool const is_mat =
true;
216 static bool const is_quat =
false;
225 template <
typename T, precision P>
226 struct type<tquat, T, P>
228 static bool const is_vec =
false;
229 static bool const is_mat =
false;
230 static bool const is_quat =
true;
237 template <
typename T, precision P>
238 struct type<tdualquat, T, P>
240 static bool const is_vec =
false;
241 static bool const is_mat =
false;
242 static bool const is_quat =
true;
252 #include "type_trait.inl"