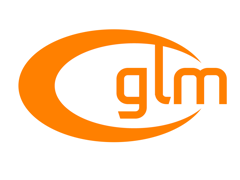 |
0.9.8
|
Go to the documentation of this file.
252 #if(GLM_COMPILER & GLM_COMPILER_GCC)
254 # if(GLM_COMPILER >= GLM_COMPILER_GCC43)
255 # define GLM_CXX11_STATIC_ASSERT
258 #elif(GLM_COMPILER & GLM_COMPILER_CLANG)
259 # if(__has_feature(cxx_exceptions))
260 # define GLM_CXX98_EXCEPTIONS
263 # if(__has_feature(cxx_rtti))
264 # define GLM_CXX98_RTTI
267 # if(__has_feature(cxx_access_control_sfinae))
268 # define GLM_CXX11_ACCESS_CONTROL_SFINAE
271 # if(__has_feature(cxx_alias_templates))
272 # define GLM_CXX11_ALIAS_TEMPLATE
275 # if(__has_feature(cxx_alignas))
276 # define GLM_CXX11_ALIGNAS
279 # if(__has_feature(cxx_attributes))
280 # define GLM_CXX11_ATTRIBUTES
283 # if(__has_feature(cxx_constexpr))
284 # define GLM_CXX11_CONSTEXPR
287 # if(__has_feature(cxx_decltype))
288 # define GLM_CXX11_DECLTYPE
291 # if(__has_feature(cxx_default_function_template_args))
292 # define GLM_CXX11_DEFAULT_FUNCTION_TEMPLATE_ARGS
295 # if(__has_feature(cxx_defaulted_functions))
296 # define GLM_CXX11_DEFAULTED_FUNCTIONS
299 # if(__has_feature(cxx_delegating_constructors))
300 # define GLM_CXX11_DELEGATING_CONSTRUCTORS
303 # if(__has_feature(cxx_deleted_functions))
304 # define GLM_CXX11_DELETED_FUNCTIONS
307 # if(__has_feature(cxx_explicit_conversions))
308 # define GLM_CXX11_EXPLICIT_CONVERSIONS
311 # if(__has_feature(cxx_generalized_initializers))
312 # define GLM_CXX11_GENERALIZED_INITIALIZERS
315 # if(__has_feature(cxx_implicit_moves))
316 # define GLM_CXX11_IMPLICIT_MOVES
319 # if(__has_feature(cxx_inheriting_constructors))
320 # define GLM_CXX11_INHERITING_CONSTRUCTORS
323 # if(__has_feature(cxx_inline_namespaces))
324 # define GLM_CXX11_INLINE_NAMESPACES
327 # if(__has_feature(cxx_lambdas))
328 # define GLM_CXX11_LAMBDAS
331 # if(__has_feature(cxx_local_type_template_args))
332 # define GLM_CXX11_LOCAL_TYPE_TEMPLATE_ARGS
335 # if(__has_feature(cxx_noexcept))
336 # define GLM_CXX11_NOEXCEPT
339 # if(__has_feature(cxx_nonstatic_member_init))
340 # define GLM_CXX11_NONSTATIC_MEMBER_INIT
343 # if(__has_feature(cxx_nullptr))
344 # define GLM_CXX11_NULLPTR
347 # if(__has_feature(cxx_override_control))
348 # define GLM_CXX11_OVERRIDE_CONTROL
351 # if(__has_feature(cxx_reference_qualified_functions))
352 # define GLM_CXX11_REFERENCE_QUALIFIED_FUNCTIONS
355 # if(__has_feature(cxx_range_for))
356 # define GLM_CXX11_RANGE_FOR
359 # if(__has_feature(cxx_raw_string_literals))
360 # define GLM_CXX11_RAW_STRING_LITERALS
363 # if(__has_feature(cxx_rvalue_references))
364 # define GLM_CXX11_RVALUE_REFERENCES
367 # if(__has_feature(cxx_static_assert))
368 # define GLM_CXX11_STATIC_ASSERT
371 # if(__has_feature(cxx_auto_type))
372 # define GLM_CXX11_AUTO_TYPE
375 # if(__has_feature(cxx_strong_enums))
376 # define GLM_CXX11_STRONG_ENUMS
379 # if(__has_feature(cxx_trailing_return))
380 # define GLM_CXX11_TRAILING_RETURN
383 # if(__has_feature(cxx_unicode_literals))
384 # define GLM_CXX11_UNICODE_LITERALS
387 # if(__has_feature(cxx_unrestricted_unions))
388 # define GLM_CXX11_UNRESTRICTED_UNIONS
391 # if(__has_feature(cxx_user_literals))
392 # define GLM_CXX11_USER_LITERALS
395 # if(__has_feature(cxx_variadic_templates))
396 # define GLM_CXX11_VARIADIC_TEMPLATES
399 #endif//(GLM_COMPILER & GLM_COMPILER_CLANG)