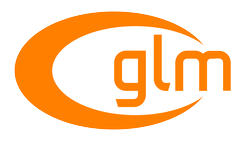 |
0.9.9 API documenation
|
Go to the documentation of this file. 15 #ifndef GLM_ENABLE_EXPERIMENTAL 16 # error "GLM: GLM_GTX_type_trait is an experimental extension and may change in the future. Use #define GLM_ENABLE_EXPERIMENTAL before including it, if you really want to use it." 20 #include "../detail/qualifier.hpp" 21 #include "../gtc/quaternion.hpp" 22 #include "../gtx/dual_quaternion.hpp" 24 #if GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED) 25 # pragma message("GLM: GLM_GTX_type_trait extension included") 36 static bool const is_vec =
false;
37 static bool const is_mat =
false;
38 static bool const is_quat =
false;
39 static length_t
const components = 0;
40 static length_t
const cols = 0;
41 static length_t
const rows = 0;
44 template<length_t L,
typename T, qualifier Q>
45 struct type<vec<L, T, Q> >
47 static bool const is_vec =
true;
48 static bool const is_mat =
false;
49 static bool const is_quat =
false;
50 static length_t
const components = L;
53 template<length_t C, length_t R,
typename T, qualifier Q>
54 struct type<mat<C, R, T, Q> >
56 static bool const is_vec =
false;
57 static bool const is_mat =
true;
58 static bool const is_quat =
false;
59 static length_t
const components = C;
60 static length_t
const cols = C;
61 static length_t
const rows = R;
64 template<
typename T, qualifier Q>
65 struct type<qua<T, Q> >
67 static bool const is_vec =
false;
68 static bool const is_mat =
false;
69 static bool const is_quat =
true;
70 static length_t
const components = 4;
73 template<
typename T, qualifier Q>
74 struct type<tdualquat<T, Q> >
76 static bool const is_vec =
false;
77 static bool const is_mat =
false;
78 static bool const is_quat =
true;
79 static length_t
const components = 8;
85 #include "type_trait.inl"