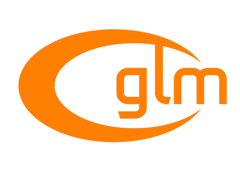 |
0.9.7
|
Go to the documentation of this file.
44 #if !GLM_HAS_CXX11_STL
45 # error "GLM_GTX_hash requires C++11 standard library support"
50 #include "../vec2.hpp"
51 #include "../vec3.hpp"
52 #include "../vec4.hpp"
53 #include "../gtc/vec1.hpp"
55 #include "../gtc/quaternion.hpp"
56 #include "../gtx/dual_quaternion.hpp"
58 #include "../mat2x2.hpp"
59 #include "../mat2x3.hpp"
60 #include "../mat2x4.hpp"
62 #include "../mat3x2.hpp"
63 #include "../mat3x3.hpp"
64 #include "../mat3x4.hpp"
66 #include "../mat4x2.hpp"
67 #include "../mat4x3.hpp"
68 #include "../mat4x4.hpp"
72 template <
typename T, glm::precision P>
73 struct hash<
glm::tvec1<T,P>>
75 GLM_FUNC_DECL
size_t operator()(
const glm::tvec1<T,P> &v)
const;
78 template <
typename T, glm::precision P>
79 struct hash<
glm::tvec2<T,P>>
81 GLM_FUNC_DECL
size_t operator()(
const glm::tvec2<T,P> &v)
const;
84 template <
typename T, glm::precision P>
85 struct hash<
glm::tvec3<T,P>>
87 GLM_FUNC_DECL
size_t operator()(
const glm::tvec3<T,P> &v)
const;
90 template <
typename T, glm::precision P>
91 struct hash<
glm::tvec4<T,P>>
93 GLM_FUNC_DECL
size_t operator()(
const glm::tvec4<T,P> &v)
const;
96 template <
typename T, glm::precision P>
97 struct hash<
glm::tquat<T,P>>
99 GLM_FUNC_DECL
size_t operator()(
const glm::tquat<T,P> &q)
const;
102 template <
typename T, glm::precision P>
103 struct hash<
glm::tdualquat<T,P>>
105 GLM_FUNC_DECL
size_t operator()(
const glm::tdualquat<T,P> &q)
const;
108 template <
typename T, glm::precision P>
109 struct hash<
glm::tmat2x2<T,P>>
111 GLM_FUNC_DECL
size_t operator()(
const glm::tmat2x2<T,P> &m)
const;
114 template <
typename T, glm::precision P>
115 struct hash<
glm::tmat2x3<T,P>>
117 GLM_FUNC_DECL
size_t operator()(
const glm::tmat2x3<T,P> &m)
const;
120 template <
typename T, glm::precision P>
121 struct hash<
glm::tmat2x4<T,P>>
123 GLM_FUNC_DECL
size_t operator()(
const glm::tmat2x4<T,P> &m)
const;
126 template <
typename T, glm::precision P>
127 struct hash<
glm::tmat3x2<T,P>>
129 GLM_FUNC_DECL
size_t operator()(
const glm::tmat3x2<T,P> &m)
const;
132 template <
typename T, glm::precision P>
133 struct hash<
glm::tmat3x3<T,P>>
135 GLM_FUNC_DECL
size_t operator()(
const glm::tmat3x3<T,P> &m)
const;
138 template <
typename T, glm::precision P>
139 struct hash<
glm::tmat3x4<T,P>>
141 GLM_FUNC_DECL
size_t operator()(
const glm::tmat3x4<T,P> &m)
const;
144 template <
typename T, glm::precision P>
145 struct hash<
glm::tmat4x2<T,P>>
147 GLM_FUNC_DECL
size_t operator()(
const glm::tmat4x2<T,P> &m)
const;
150 template <
typename T, glm::precision P>
151 struct hash<
glm::tmat4x3<T,P>>
153 GLM_FUNC_DECL
size_t operator()(
const glm::tmat4x3<T,P> &m)
const;
156 template <
typename T, glm::precision P>
157 struct hash<
glm::tmat4x4<T,P>>
159 GLM_FUNC_DECL
size_t operator()(
const glm::tmat4x4<T,P> &m)
const;